By: Team F10-3
Since: Aug 2019
Licence: MIT
1. Setting up
Refer to the guide here.
2. Design
2.1. Architecture
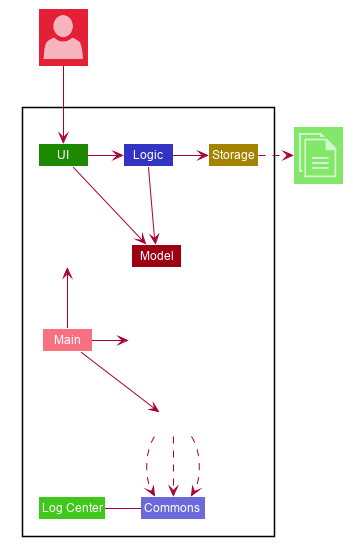
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.
The .puml files used to create diagrams in this document can be found in the diagrams folder.
Refer to the Using PlantUML guide to learn how to create and edit diagrams.
|
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components.
The following class plays an important role at the architecture level:
-
LogsCenter
: Used by many classes to write log messages to the App’s log file.
The rest of the App consists of four components.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (see the class diagram given below) defines it’s API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.

How the architecture components interact with each other
The Sequence Diagram below shows how the components interact with each other for the scenario where the user issues the command delete 1
.
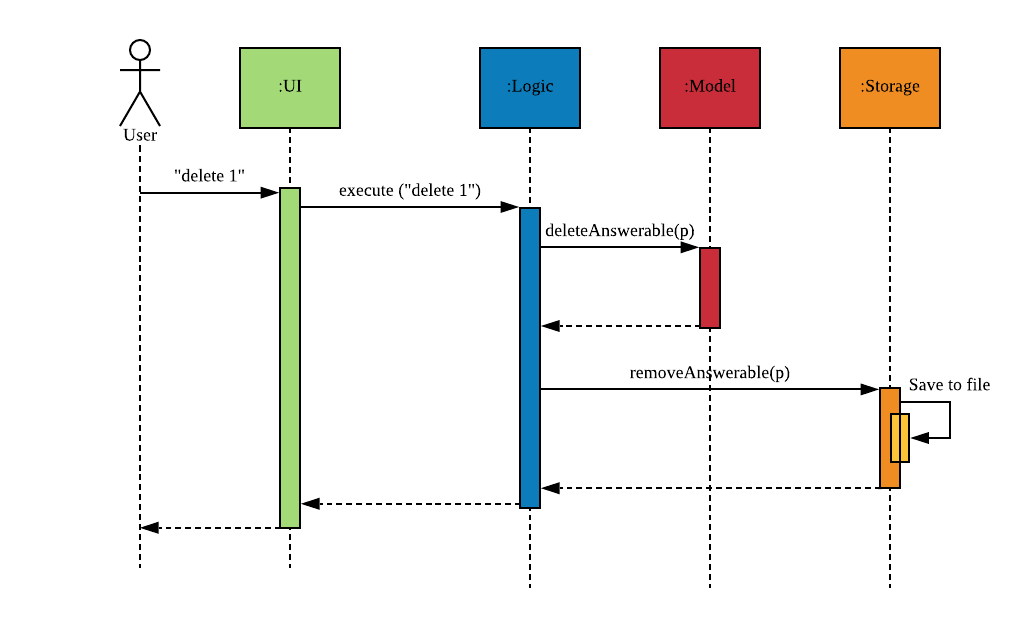
delete 1
commandThe sections below give more details of each component.
2.2. UI component
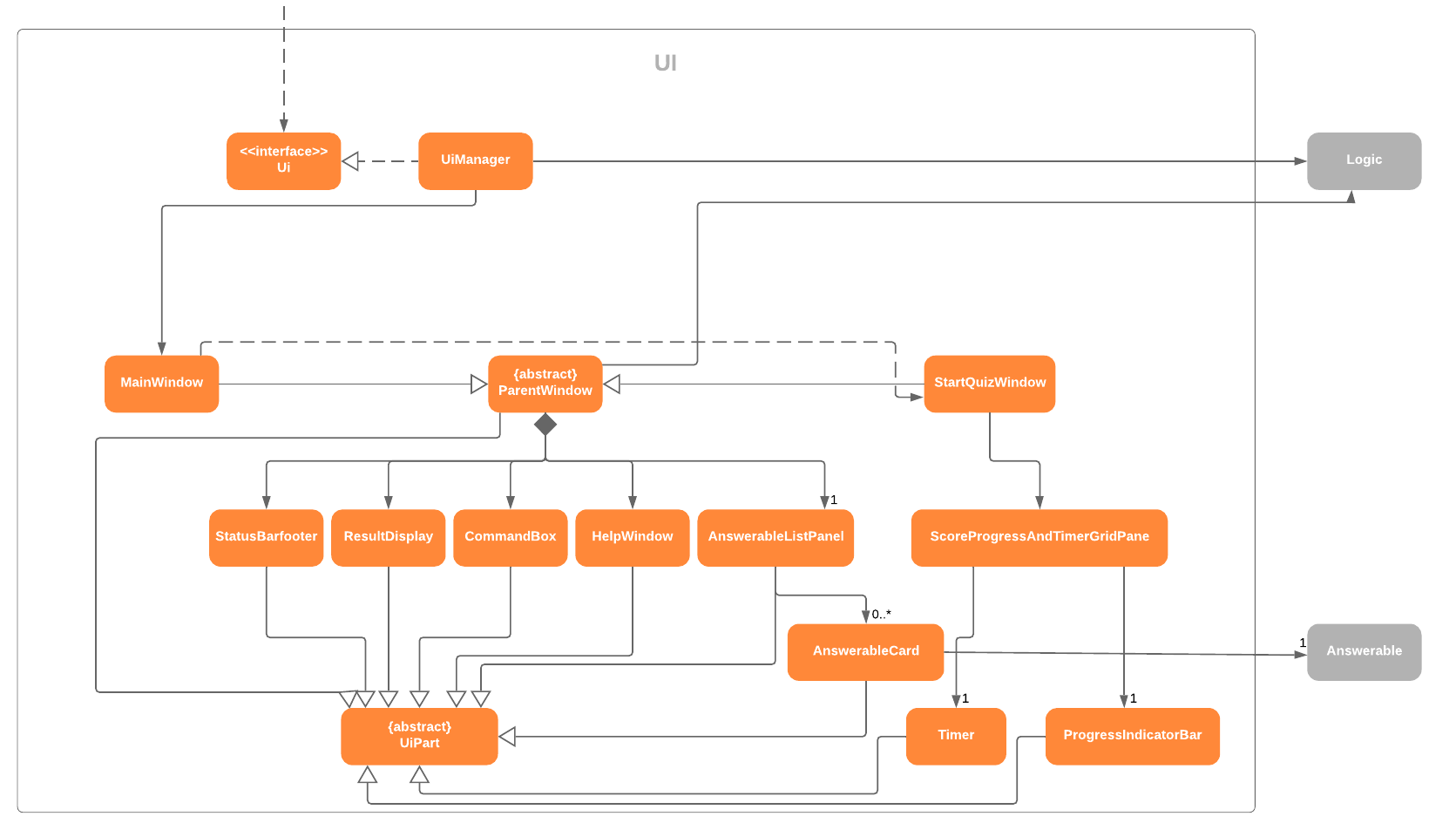
API : Ui.java
The abstract class ParentWindow
consists of individual Ui parts e.g.CommandBox
, ResultDisplay
, AnswerableListPanel
, StatusBarFooter
etc. All Ui classes inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The MainWindow
inherits from the ParentWindow
class and handles the display of information in the configuration mode. Key responsibilities of the MainWindow
include
-
Execute user commands through the
CommandBox
using theLogic
component. -
Listen for changes to
Model
data so that the UI can be updated to reflect the modified data. This occurs for two types of situations-
Direct modification to the information inside the Answerables List, such as
AddCommand
orEditCommand
-
Filtering of the currently shown list, for commands such as
FindCommand
andListCommand
-
The StartQuizWindow
inherits from the ParentWindow
class and handles the display of information during quiz mode. It has an additional ScoreProgressAndTimerGridPane
Ui component, which
is used to contain score progress and timer related Ui. Key responsibilities of the StartQuizWindow
include
-
Execute user answer input, e.g. "A", "B", "C", "D" for
Mcq
-
Adapt to changes in the current
Answerable
and update the Ui accordingly based on a few typical situations-
from the four options of
Mcq
to the two options forTrueFalse
-
update the progress bar for every
Answerable
-
update the timer every second and switch to the next
Answerable
when countdown reaches 0.
-
2.2.1. Design Considerations
Aspect: Implementation of the Ui for both windows
-
Alternative 1 (current choice): Have a parent class
ParentWindow
which is extended byMainWindow
andStartQuizWindow
-
Pro1: Adheres to the Single Responsibility Principle, where the
MainWindow
only has one reason to change, and changes in quiz mode should not affect theMainWindow
-
Pro2: The abstract
ParentWindow
class follows the Open/Closed Principle, where theStartQuizWindow
extends upon the Ui components and adds it’s own Timer and ProgressBar Ui component. Each class is also able to have their own implementation of theexecuteCommand(String commandText)
method. -
Con: Dependency between
MainWindow
andStartQuizWindow
classes in the methodsMainWindow#handleStart
andStartQuizWindow#handleEnd
respectively
-
-
Alternative 2 (initial choice): Handle all user commands and changes in Ui within the MainWindow.
-
Pro: Less overall code, quiz mode only needs to edit the content in the
AnswerableListPanel
. -
Con: As the CommandBox is a functional interface, it can only take in one abstract method as a parameter. This would mean that
MainWindow#executeCommand
would need to handle all cases of user inputs, for both answerable input commands and configuration mode commands. TheMainWindow#executeCommand
would be very long with complicated logic, thus violating SLAP.
-
2.3. Logic component
2.3.1. Overview of Logic Component
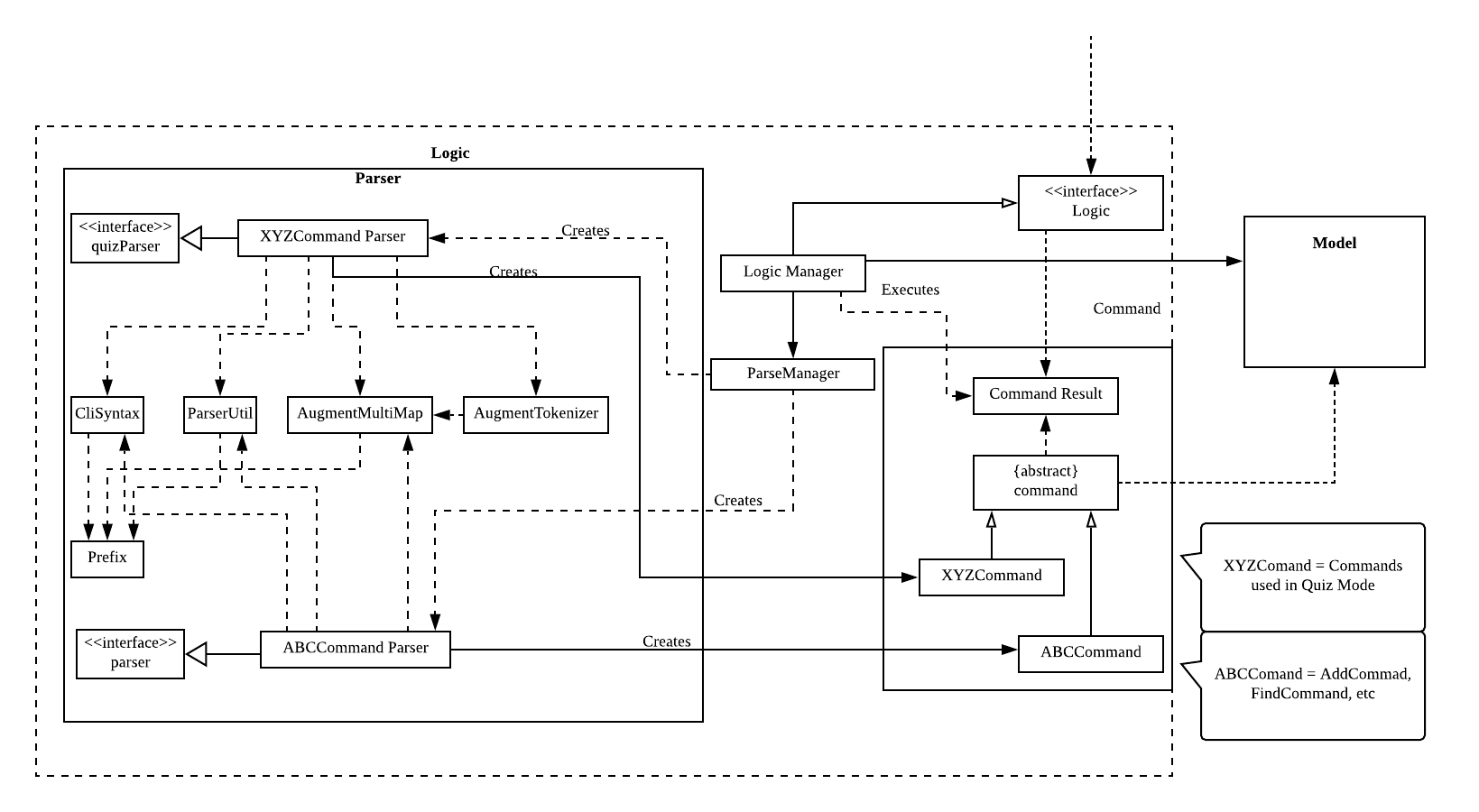
API :
Logic.java
-
Logic
uses theParserManager
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding anAnswerable
). -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
. -
In addition, the
CommandResult
object can also instruct theUi
to perform certain actions, such as displaying help to the user. -
In quiz mode, the
CommandResult
object is also used to determine whether the user’s answer is correct.
2.3.2. Managing parsing in Configuration and Quiz Mode (Overview)
The Revision Tool uses two Parser interfaces (Parser
and QuizParser
) to parse different sets of commands (i.e. in Configuration Mode and in Quiz Mode).
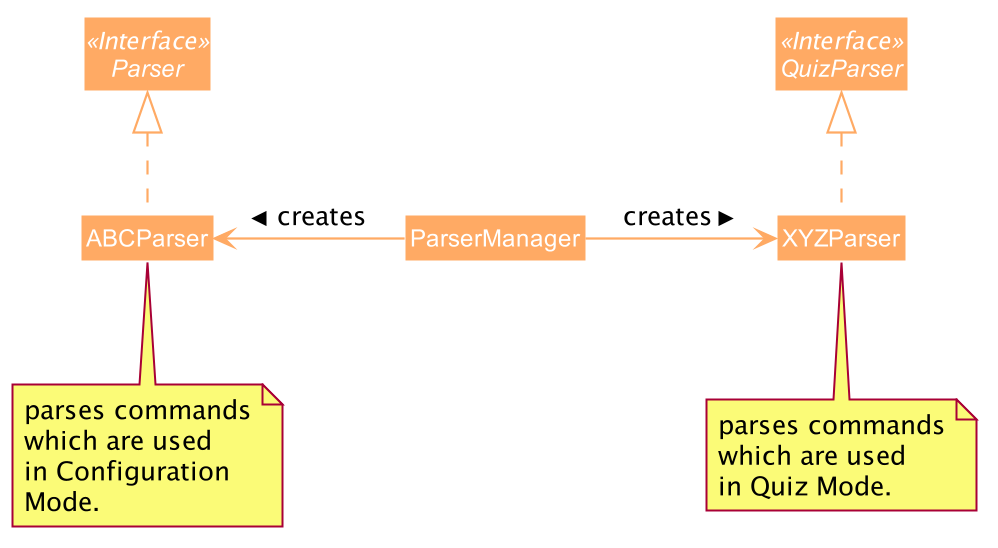
As shown in the figure above, the ParserManager
class is responsible for creating the respective parsers for Configuration and Quiz Mode.
This was designed while taking into consideration that the Quiz Mode Parsers (i.e. XYZParsers) will require
an extra Answerable
argument on top of the user input String in order to carry out commands such as determining whether the user’s input is the correct answer.
(E.g. to call methods such as Answerable#isCorrect(Answer))
As different commands are accepted in Configuration and Quiz Mode, the ParserManager
class uses overloaded methods
(parseCommand(String)
and parseCommand(String, Answerable)
to determine the valid commands in each mode. If a Configuration Mode
command such as add
were to be used in Quiz Mode, the ParserManager would deem the the command as invalid.
With reference to Figure 6, The following are the parsers used in each mode:
-
ABCParser (Configuration Mode):
-
AddCommandParser
-
DeleteCommandParser
-
EditCommandParser
-
FindCommandParser
-
ListCommandParser
-
StartCommandParser
-
-
XYZParser (Quiz Mode):
-
McqInputCommandParser
-
TfInputCommandParser
-
SaqInputCommandParser
-
A more detailed description of the implementation of parsing in Configuration and Quiz Mode and its design considerations can be found in Section 3.2, “Configuration and Quiz Mode”.
2.4. Model component
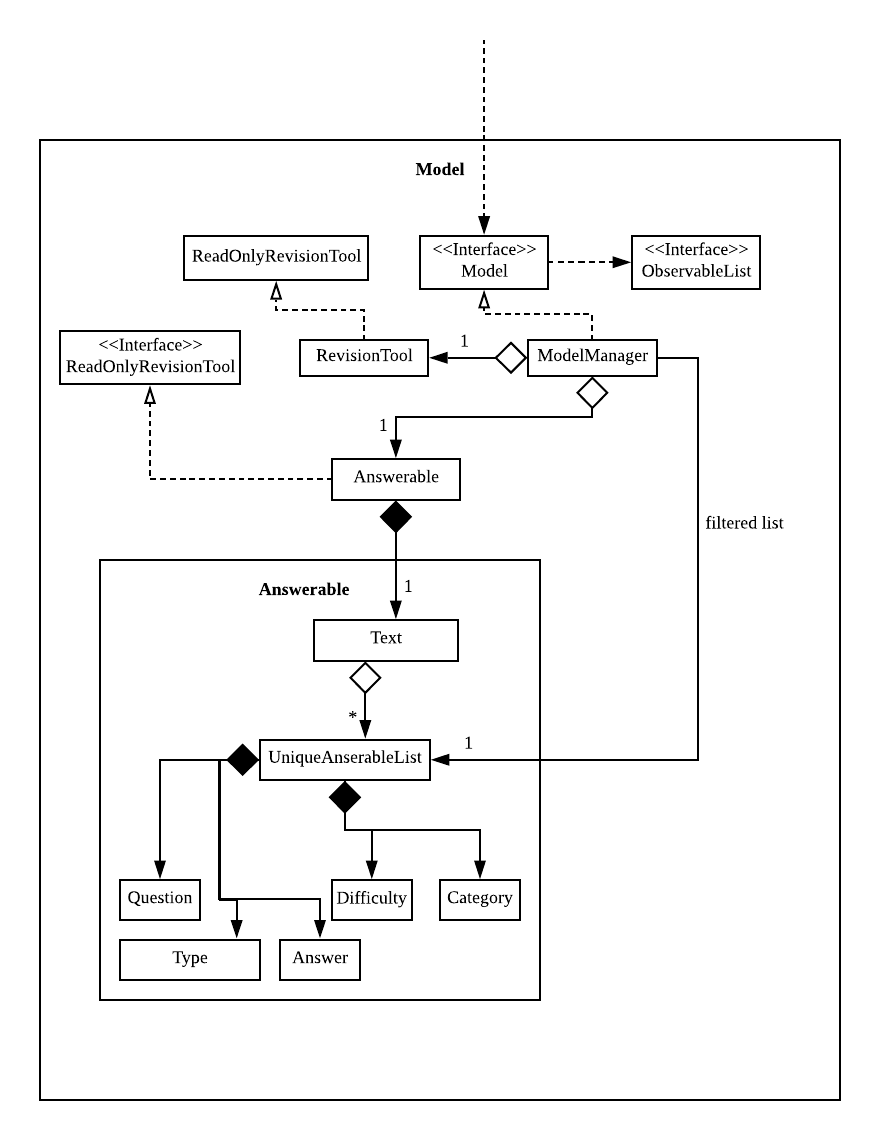
API : Model.java
The Model
,
-
stores a
QuestionBank
object that represents theQuestionBank
. -
stores the
Question Bank
data. -
exposes an unmodifiable
ObservableList<Answerable>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
does not depend on any of the other three components.
2.4.1. The Answerable
Class
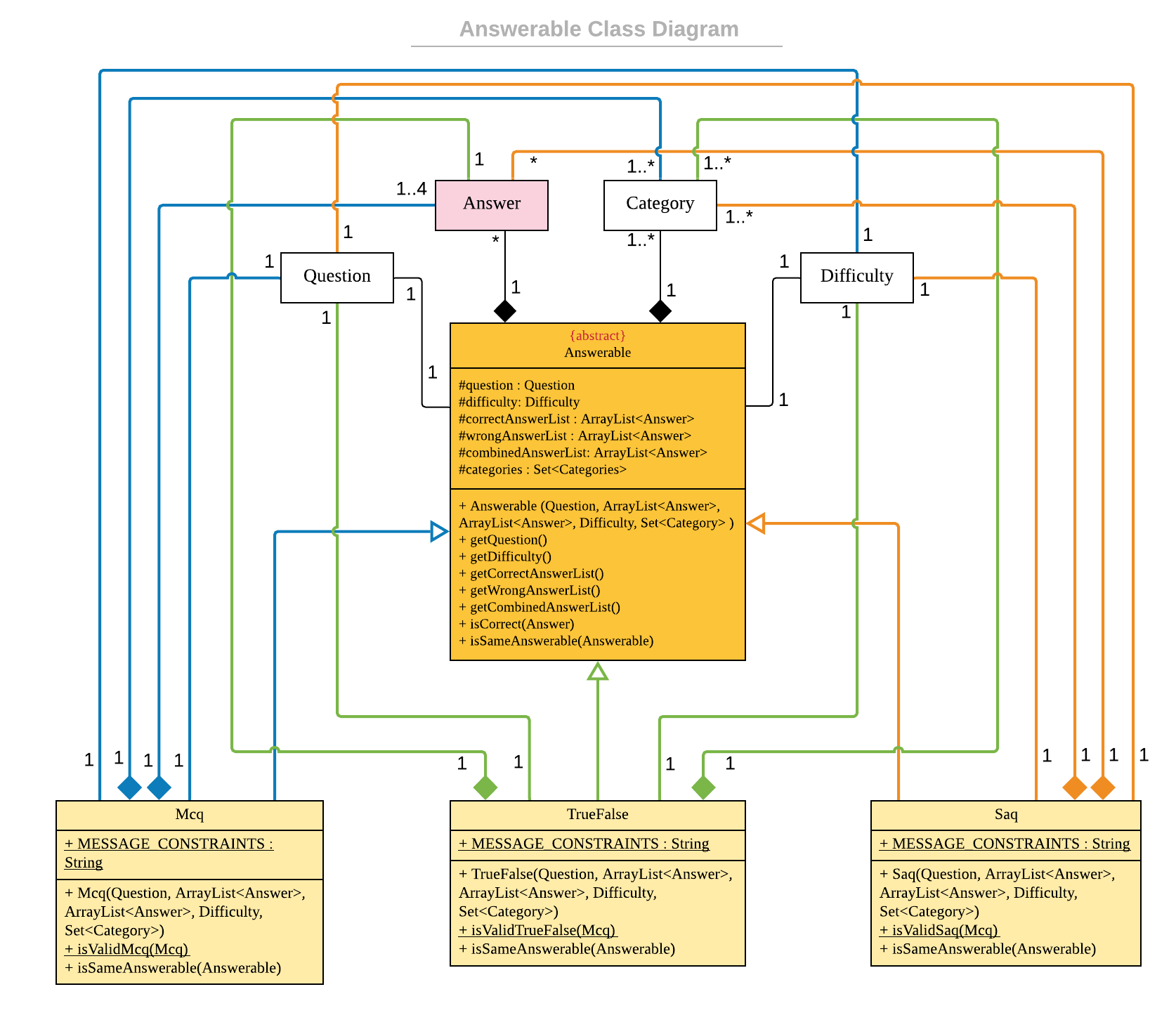
The main class that the Revision Tool operates on is the Answerable
class.
Each Answerable
class must have 1 Answerable
, 1 Difficulty
and can have any amount of categories associated with it.
The amount of answers that an Answerable
can have depends on its type.
There are 3 subclasses of the Answerable
Class which are: Mcq
, TrueFalse
and Saq
. Each class defines it’s
own rules on the validity of Answer
s (highlighted in red in the class diagram) provided to it.
The following are the rules of validity for each subclass:
-
Mcq: 4 answers in total. 1 correct answer, 3 wrong answers.
-
TrueFalse: Either 'true' or 'false' as its answer.
-
Saq: Any amount of answers.
For all subclasses, there cannot be any duplicates of answers. For example, if an Mcq class has "option1" as one of its wrong answers, it cannot have "option1" as its correct answer or another wrong answer.
2.5. Storage component
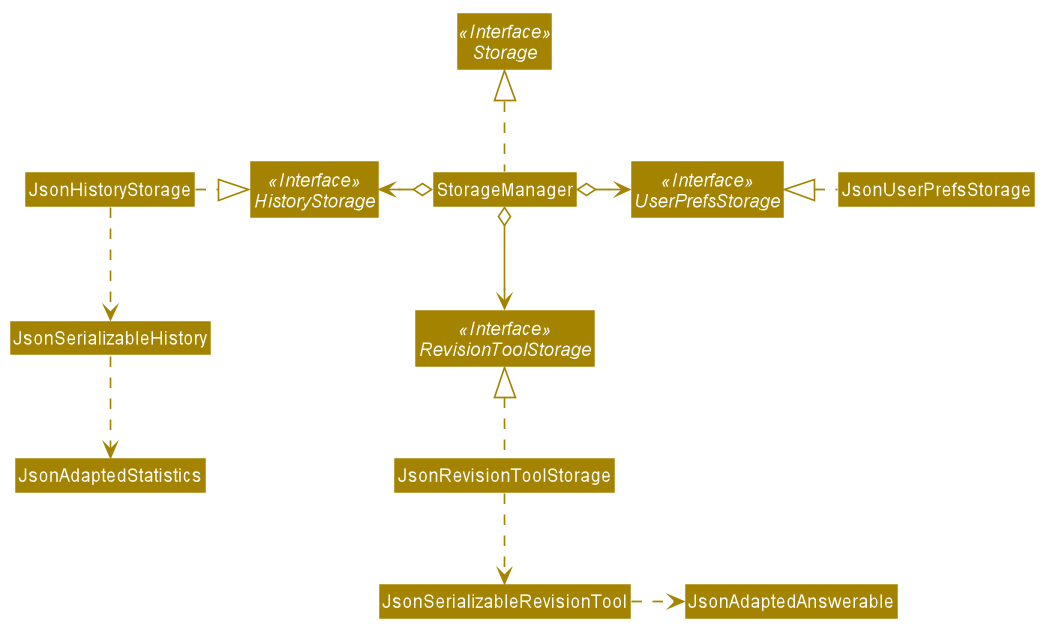
API : Storage.java
The Storage
component,
-
can save
Answerable
objects in json format and read it back. -
can save the
Statistics
data in json format and read it back.
2.6. Common classes
Classes used by multiple components are in the seedu.revision.commons
package.
3. Implementation
This section describes some noteworthy details on how certain features are implemented.
3.1. Add Answerable
feature
3.1.1. Implementation
The add Answerable`s mechanism is facilitated by `AddCommand
.
It extends Command
that will read a user command and execute the command result.
Additionally, it implements the following operations:
-
AddCommand#addMcq()
— Adds a mcq question to the question bank. -
AddCommand#addShortAns()
— Adds a short answer question to the question bank. -
AddCommand#addTf()
— Adds a True False answer question to the question bank.
These operations are exposed in the Model
interface as Model#addMcqCommand()
, Model#addTfCommand()
and Model#addShortAnsCommand()
respectively.
Given below is an example usage scenario and how the add `Answerable`s mechanism behaves at each step.
Step 1. The user types add type/mcq q/"string of `Answerable`" x/option1 x/option2 y/option3 x/option4 cat/[UML] diff/[easy]
, this command adds a easy difficulty mcq Answerable
about UML with 4 options and option3 being the correct answer.
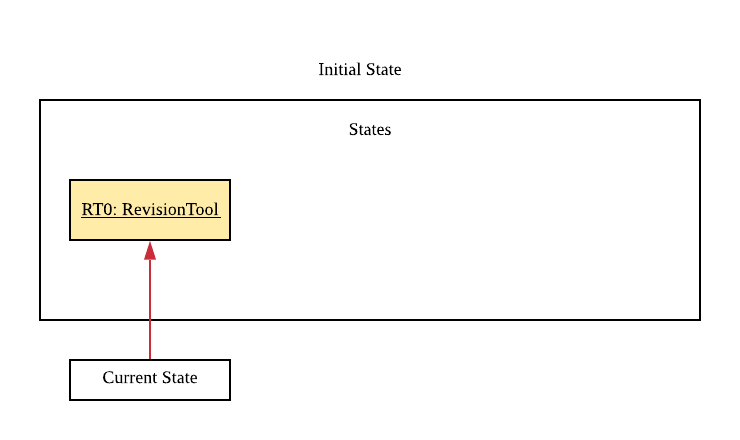
Step 2. The command is being parse into the parser and the AddCommand object of type Command will be created.
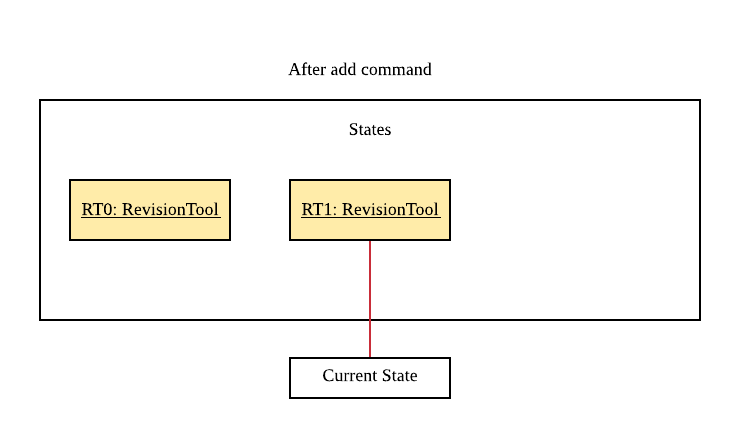
Step 3. The AddCommand object will call its addMcq()
method, this method will read the command and store the Answerable
with the answers into the test bank
accordingly.
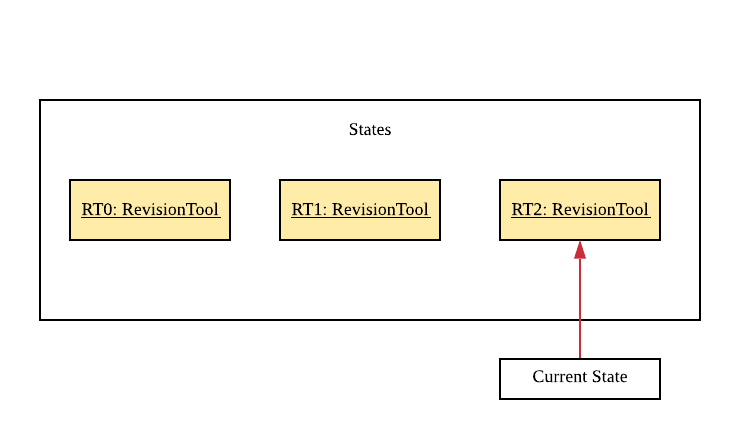
If a command fails its execution, it will not save the Answerable into the revision tool . It will however throw an invalid command exception.
|
3.1.2. Design Considerations
Aspect: How add executes
-
User enters the command "add …".
-
Command is taken in and parsed to validate if it is a valid command.
-
Add command is executed.
-
New question is saved in the question bank.
Aspect: Format of the add command
-
Use a single line containing all the information of the question.
-
Pros: Faster for user to add a question.
-
Cons: Hard for user to memorize the long sequence which may cause invalid command input.
-
Other alternative we considered: Use multiple steps to guide user on adding the question.
-
-
Pros: User do not have to memorize the correct format and less chance for an invalid command input.
-
Cons: Slow to add
Answerable`s, requiring multiple steps to fully complete an `Answerable
.
3.2. Configuration and Quiz Mode
As different commands are available for Configuration and Quiz Mode, we have to determine which commands are valid based on the state of the application. To implement this, we had to examine two main design considerations: The structure of the parser component and how to determine which parser to use. We will discuss these considerations in the following segment.
3.2.1. Design Considerations
Aspect |
Alternative 1 |
Alternative 2 |
Conclusion and Explanation |
Structure of the Parser Component |
Command parsers for both modes implement the same interface (i.e. implement both |
Command parsers belonging to each mode implement different interfaces (i.e. a |
Alternative 2 was implemented. The main reason for this choice was to adhere to the interface-segregation principle. If alternative 1 were to be implemented, a Configuration Mode command may have to implement a parse(String, Answerable) dummy method which it will not use. This is bad design as a client might be able to call the dummy method and receive unexpected results. Thus, by separating the interfaces, clients will only need to know about the methods that they need. |
Determining which parser to use |
Create two parser manager classes (i.e. |
Use a single |
Alternative 2 was implemented. By doing so, we were able to adopt a facade design pattern. The main benefit would be that
the client doesn’t need to know the logic involved in selecting which type of parser and logic to use. This hides the internal
complexity of the |
3.2.2. Commands in Configuration Mode
In Configuration Mode, a single string is passed as an argument to the Logic#execute method (i.e. execute(String)
).
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete 1")
API call.
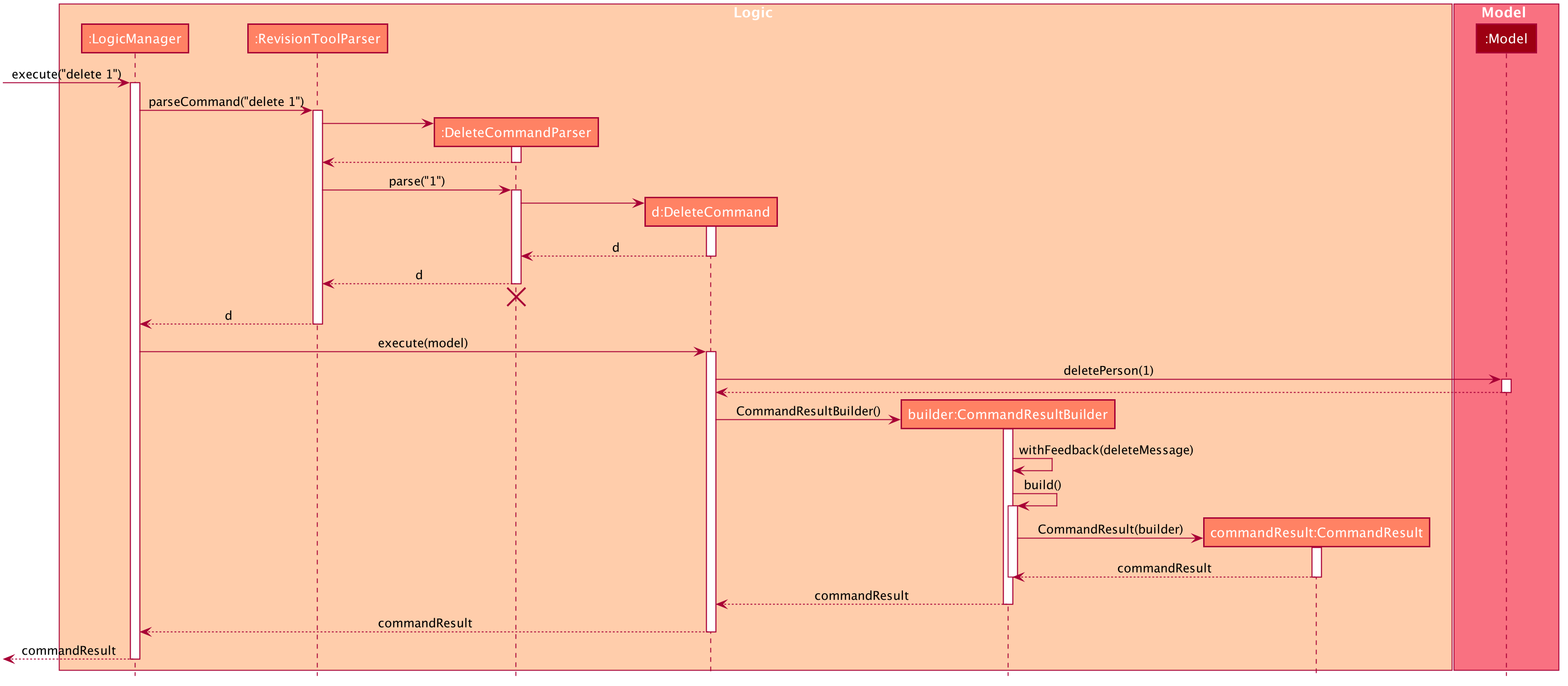
delete 1
Command
The lifeline for DeleteCommandParser should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
|
3.2.3. Commands in Quiz Mode
In Quiz Mode, a string and the current Answerable
object are passed as arguments to the Logic#execute method. (i.e. execute(String, Answerable)
)
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("c", answerable)
API call.
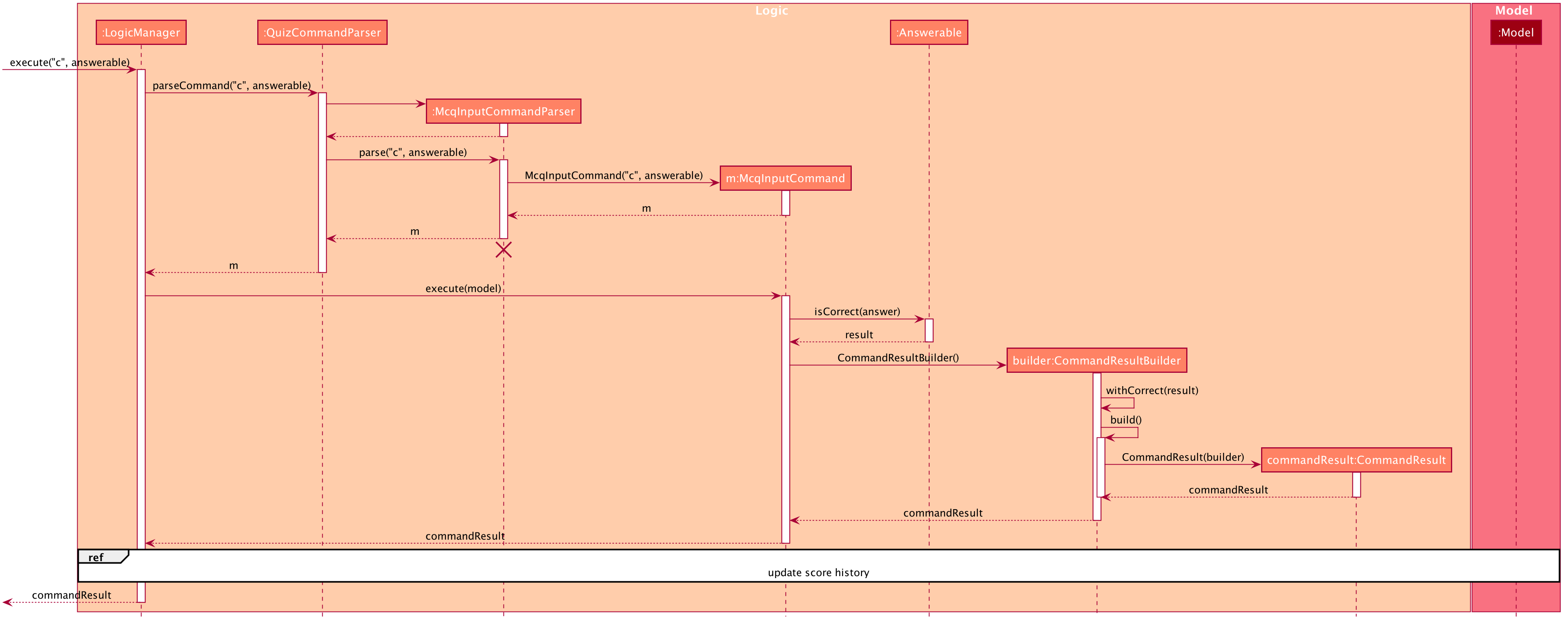
c
input command.3.2.4. Key differences between Configuration Mode and Quiz Mode:
Configuration Mode |
Quiz Mode |
Logic#execute takes in a single string. |
Logic#execute takes in a String and an Answerable. |
No methods of |
|
|
|
|
|
3.2.5. Implementation of CommandResult
(Builder Design Pattern)
The CommandResult
class is designed using a builder pattern to allow flexibility of values returned to the LogicManager
.
To guard against null values, default values are provided to every field in the CommandResult
class upon construction.
Objects that call CommandResult
can choose to customise CommandResult
according to their needs.
Below is a code snippet of the CommandResultBuilder
and CommandResult
class:
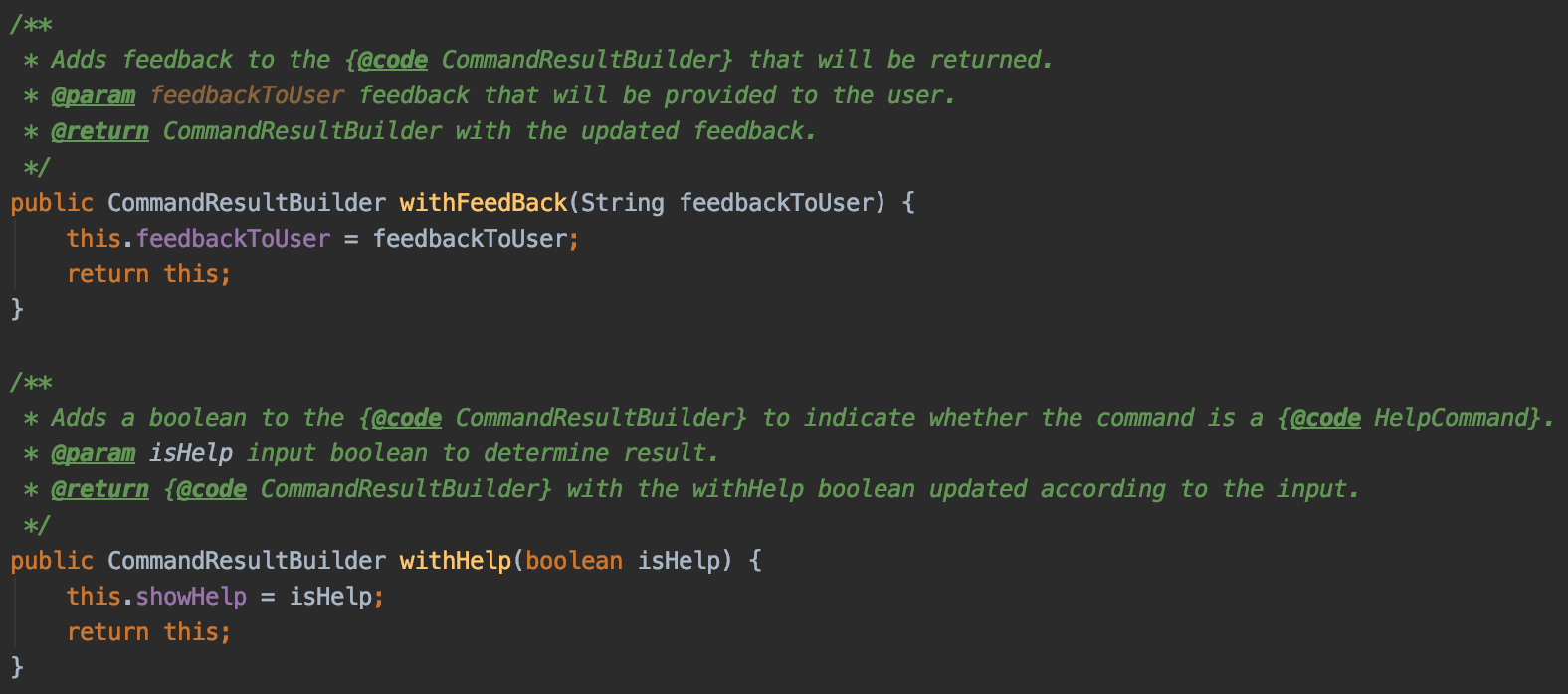
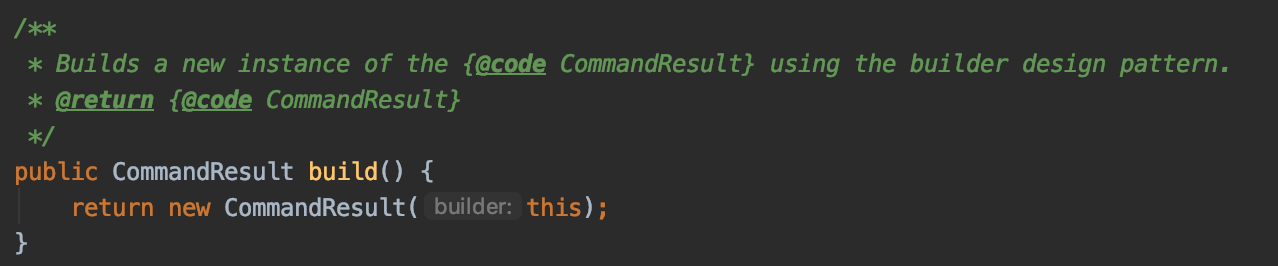
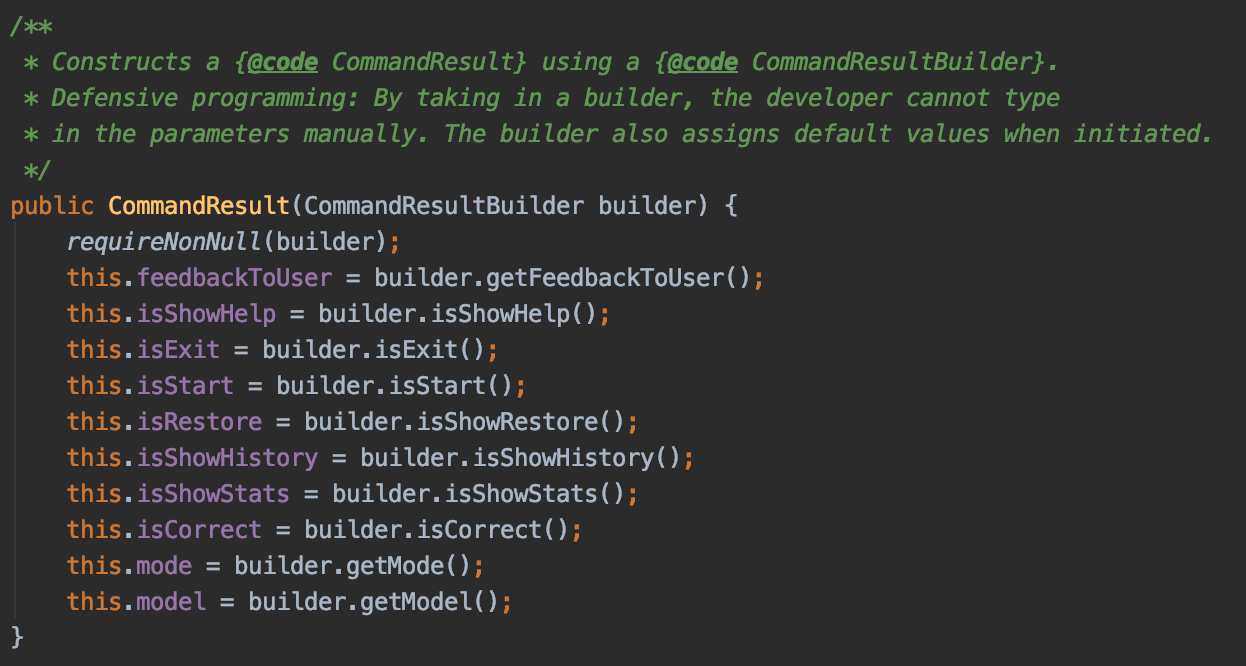
Examples of how to build a CommandResult:
CommandResult c = new CommandResultBuilder().withFeedback(message).withExit(true).build(); CommandResult c = new CommandResultBuilder().isCorrect(true).build();
3.2.6. How the quiz works
After the user has start
ed the quiz, the application enters Quiz Mode. The following is the flow of events after a quiz
session has started.
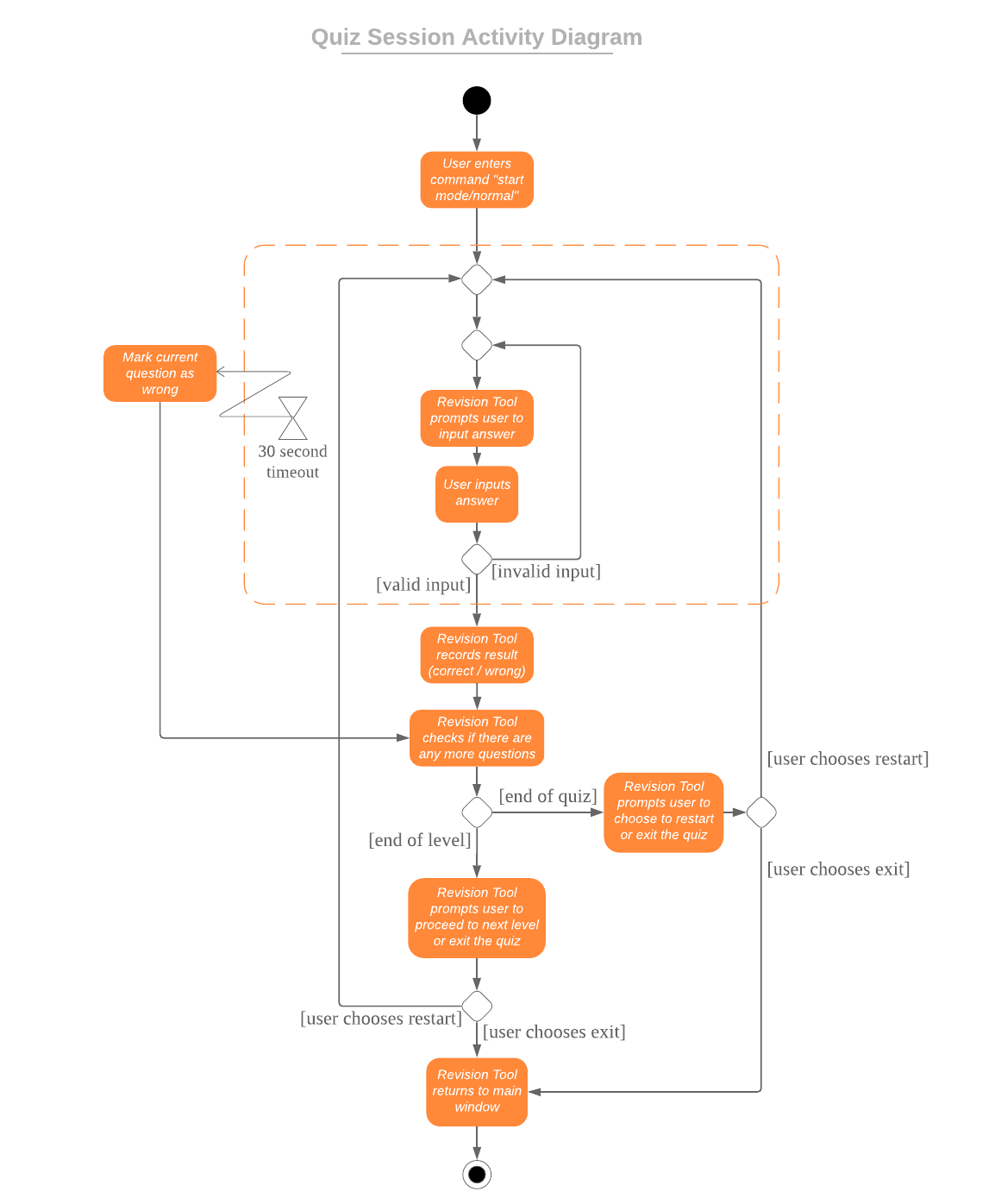
NormalMode
Elaboration of Steps:
-
After the user has started a normal mode quiz. He/she will be prompted to key in their answer.
-
If the input is valid, the revision tool will display the subsequent question until the level / entire quiz has ended.
-
If the input is invalid, the revision tool will prompt the user to key in their input again with guidance provided on the commands accepted.
-
If the time limit is exceeded (e.g. 30 seconds in Normal Mode), the revision tool will mark the
Answerable
as wrong and move on to the nextAnswerable
. -
Once a level has ended, the user will be given the choice to move on to the next level or exit the quiz.
-
Once the entire quiz has ended, the user will be given the choice to restart or exit the quiz.
For Custom Mode, the game play is the same except that user can choose the category, difficulty and timer for the questions. For Arcade Mode, when a users enters a wrong answer, the quiz will end. |
3.3. Data Analytics
3.3.1. Overview
There are many ways to present additional data to the user. While looking at user stories and deciding what our
target audience will need, we realised that one important information needs to be present - the type of questions
that user performs the weakest in. In order to present this information to the user, we needed a way to record
results of quizzes, store these results, break down these results according to question types and report the type
of questions that the user has performed badly in. These will be showcased in the stats
command feature.
3.3.2. Implementation
updateStatistics feature
Quiz results are first recorded by successful completion of quizzes in Normal mode. At each quiz attempt,
a Statistics
object is first initiated. Every correct answer parsed by the user will trigger the code snippet below
to updateStatistics
.
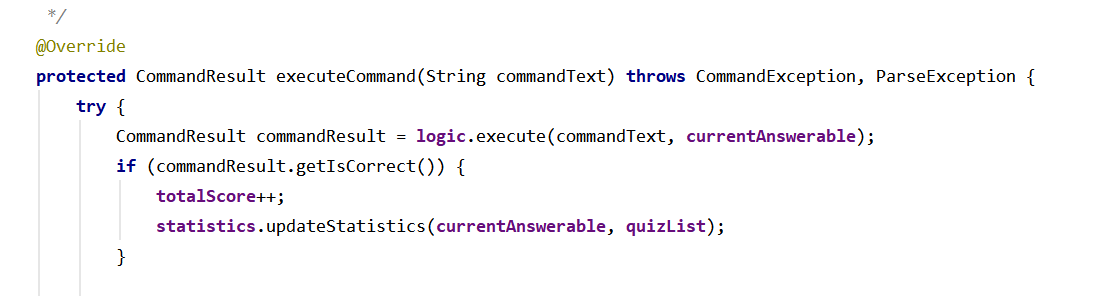
The sequence diagram for interactions between the Logic
component and the Model
component, from
parsing of a correct answer in the Logic
component to updating the Statistics
class in the Model
component is
already shown above in Figure 11
.
updateHistory feature
After the user successfully completes a quiz in Normal mode, History
will be updated with this 'Statistics' object.
History
serves as a storage component for quiz results. This is done using the code snippet shown below.
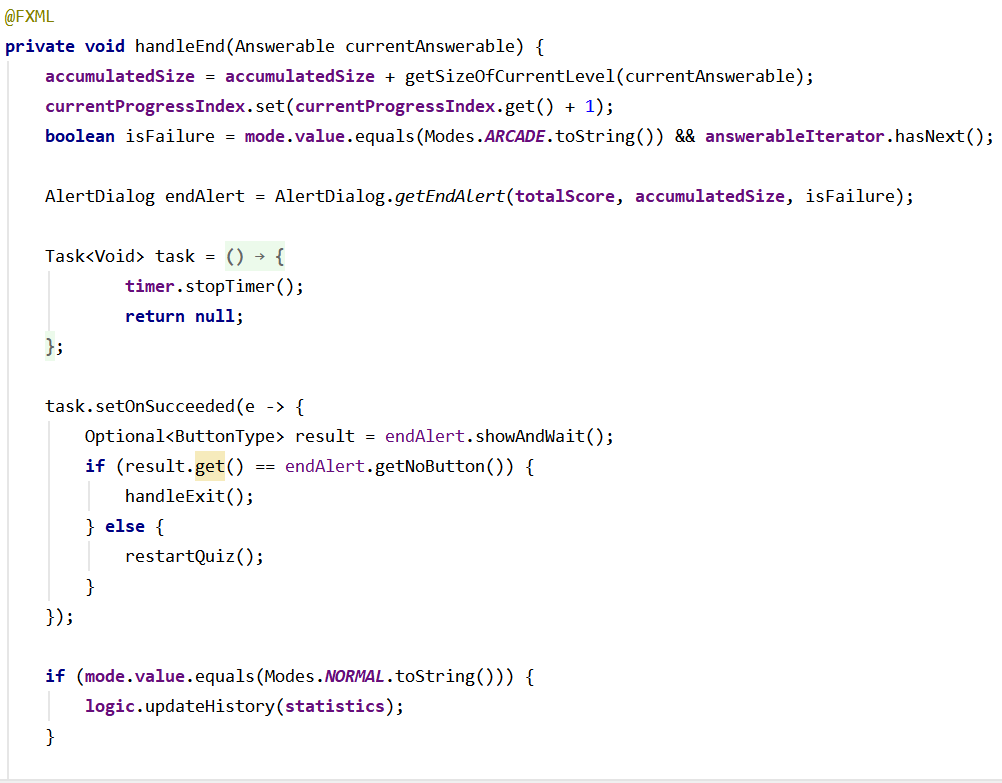
Given below is the sequence diagram for interactions between the`Ui`component, Logic
component and the
Model
component, from handling the end of a quiz in the Ui
component to updating the History
class
with the Statistics
object in the Model
component.
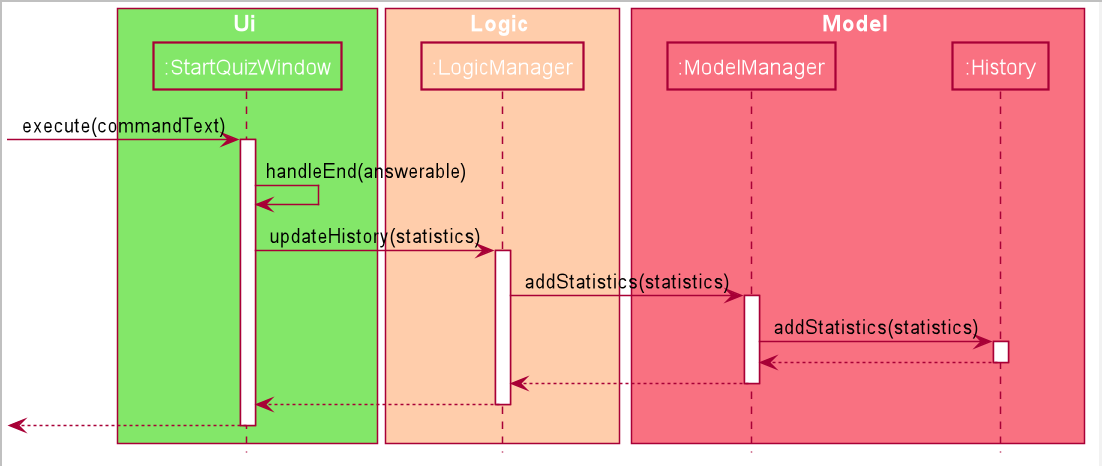
Stats Command Feature
Given below is the sequence diagram for interactions within the Logic
component for the execute("stats")
API call.
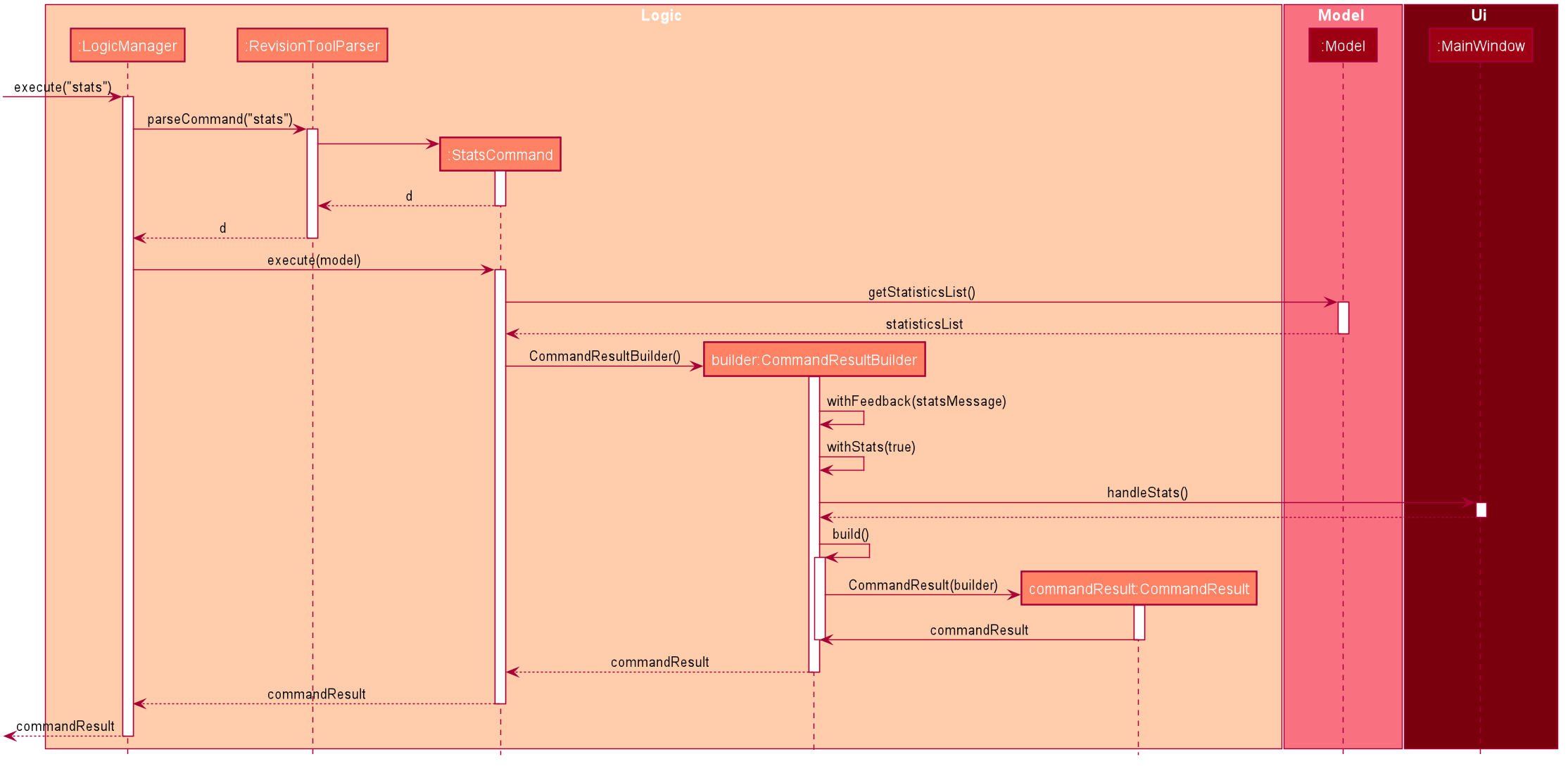
The stats command will first activated the parser in Logic
before executing the StatsCommand
.
This will then get the list of past statistics of quiz results from the model before sending a feedback
to the user through the CommandResultBuilder
. The CommandResultBuilder
will trigger the
handleStats
method to finally show the list of statistics in the MainWindow through the Ui
.
3.4. Saq feature
3.4.1. How Saq works
The Saq input mechanism is facilitated by SaqInputCommand
. It extends Command
and execute the CommandResult
. The input
will be parse into SaqInputCommandParser
to check for input validity. If the input String is not valid (i.e the String
does not start with a letter of number), an invalid command parse exception will be thrown, indicating to user that the
input String requires a letter or a number at the start of the String.
If the input is valid, SaqInputCommand
object of type Command
will be created. The execute command of the
SaqInputCommand
object will be executed. The execute command will call the isCorrect
method of Saq to check if the
user input is correct or not.
The activity diagram below shows the steps the user takes to answer an SAQ.
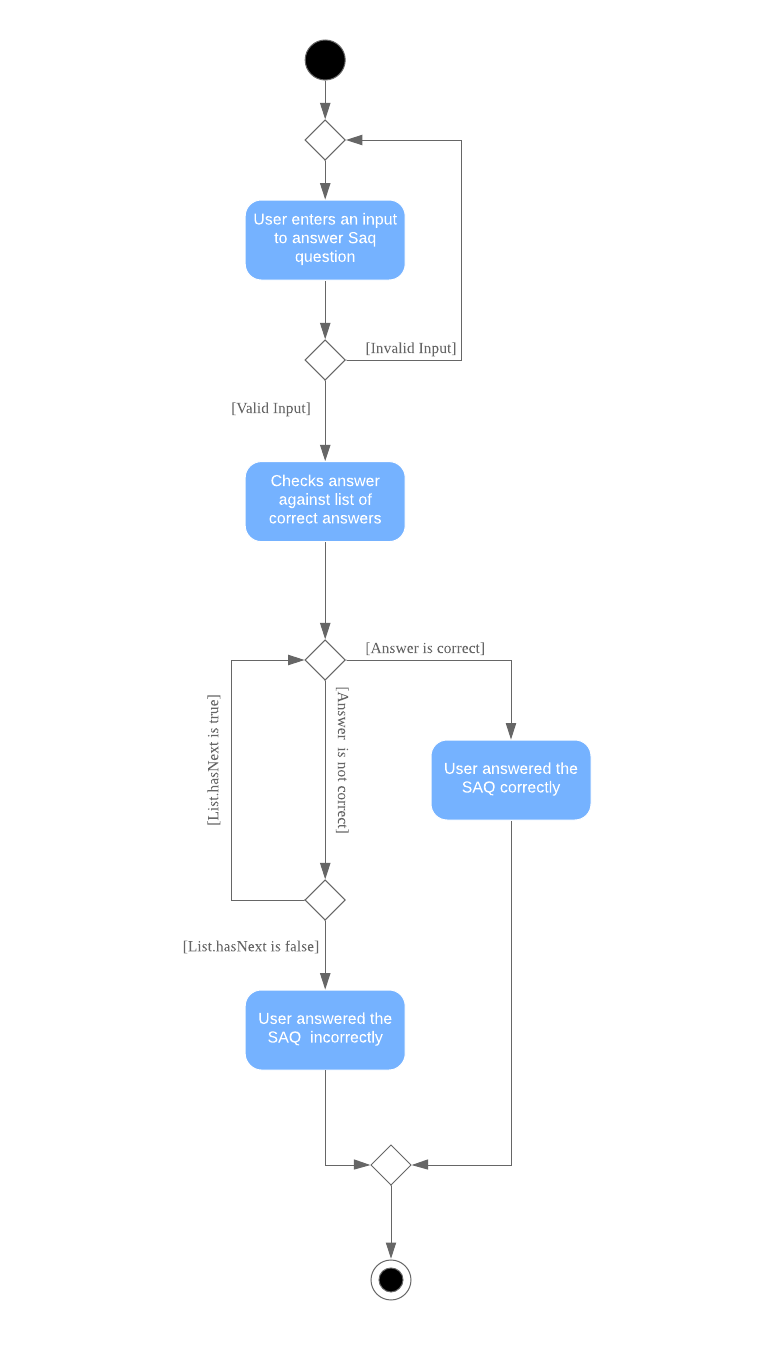
3.4.2. NLP Libraries
External libraries were used to determine if the user input is correct or not. StanfordCoreNLP
library was used to
check for sentimental value of user input and the list of correct answers. This helps to check for negative sentence
against a neutral or positive sentence (eg. "not a uml diagram" and "it’s a uml diagram"). The FuzzyWuzzy
library was
also used to determine the similarity percentage of the user input to the correct answer using fuzzy string matching
technique.
The class diagram below shows the relationship between the classes that are used to validate the correctness of the user input.
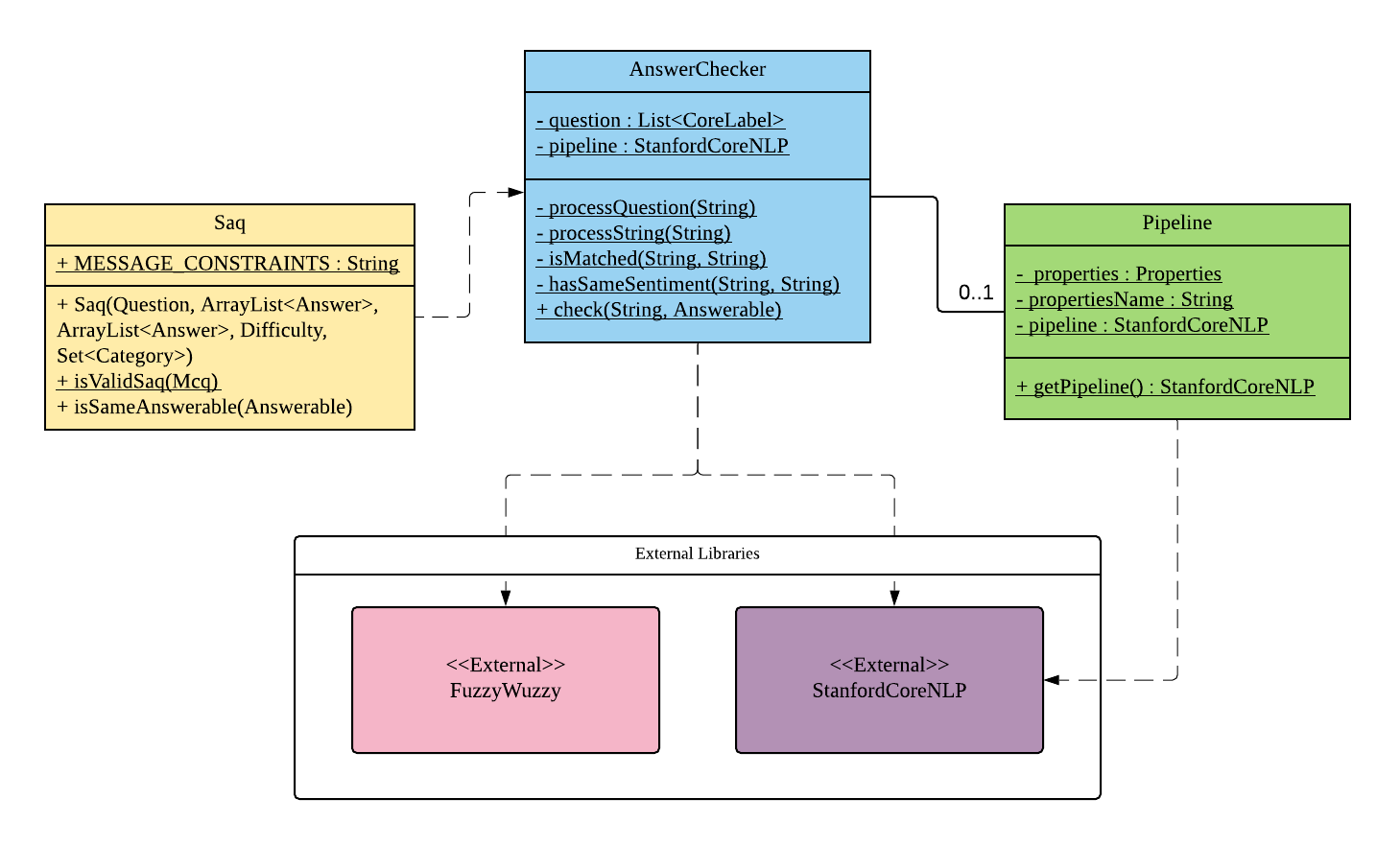
3.4.3. Validating the answer
The execute
method will return the CommandResult
, correct or wrong. The currentAnswerable of type Saq
will call its
isCorrect
method and return a boolean value, true for correct answer and false for wrong answer. The sequence diagram
below shows the steps taken by the isCorrect
method to determine if the user input is correct or wrong.
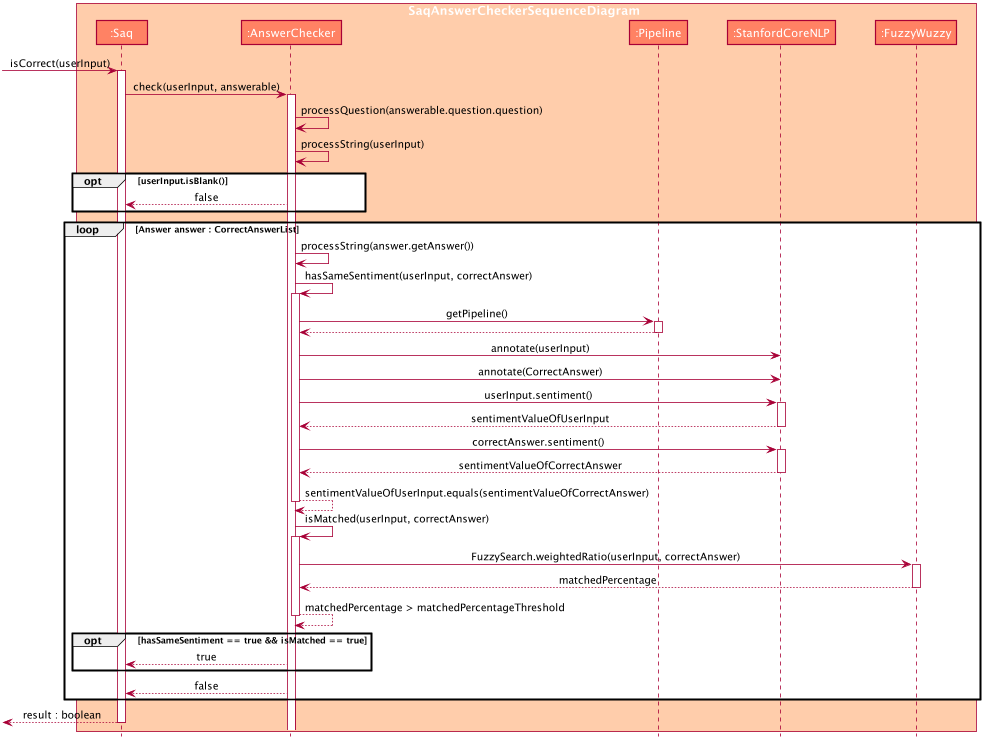
3.5. Restore feature
3.5.1. Implementation
The restore mechanism is facilitated by RestoreCommand
.
It extends Command
that will read a user command and execute the command result.
Additionally, it implements the following operations:
-
#handleRestore()
— Prompts the user with an alert box if he really wishes to execute the restore function. -
#setRevisionTool()
— Clears the current question bank and reset it with our own default questions.
These operations are exposed in the Model
interface as Model#setRevisionTool()
and from MainWindow
as #handleRestore()
respectively.
3.5.2. Design Considerations
-
When implementing the restore feature, we didn’t want users to face a problem if they entered the command accidentally hence the alert popup was implemented, to prompt users if they really want to carry out the command before executing it.
-
With this popup, users will now be more cautious when trying to restore and only do so when they really want to reset their revision tool.
-
Furthermore, the questions that we included in the default revision tool question bank are questions taken from the lecture quiz and weekly quiz which are most probably deemed important by the professor himself.
Aspect: How Restore executes
-
User enters the command "restore".
-
Command is taken in and a popup is shown to reconfirm if the user would like to carry out the restore command.
-
Upon clicking yes, restore command will be handled.
-
Current questions will be deleted and default questions will reset to the revision tool.
3.6. AutoComplete feature
3.6.1. Implementation
-
A set of commands and auto completed text are saved in a set.
-
When users type a command on the text box, method
#populatePopup
will be called where the user’s command will be matched against our SortedSet. -
If there is a match, a contextMenu showing all possible auto complete text will show up.
-
This method is implemented such that the results in the contextMenu will change and show as the user is typing and this would make it more intuitive for users.
3.6.2. Design Considerations
-
The main design consideration here would be to have value added auto complete list to pop up.
-
How we managed that is to show:
-
The basic command
-
Basic command + possible parse commands where they can easily fill in.
-

Aspect: How AutoComplete works
-
Users wishes to enter an "Add" command
add type/mcq q/What is 1 + 1 y/2 x/1 x/3 x/4 cat/easy diff/1
-
Upon typing either "a", "ad" or even "add", the auto complete context menu will pop up showing possible auto complete list, mainly:
-
add
-
add type/ q/ y/ x/ cat/ diff/
-
-
Upon seeing that, users will be able to select those options or use those as a guideline to complete his commands more intuitively.
3.7. List feature
ListCommand` extends Command
that will read in user command and execute the command result. User can filter by Catergory
and/or Difficulty
3.7.1. Design Considerations
Aspect: Showing the filtered list
-
Current Implementation
ListCommand#excute
combines theCategoryPredicate
andDifficultyPredicate
to update the answerable list throughModel#updateFilteredAnswerableList
-
Pro: Uses Java 8 streams which supports immutability. This is in line with the immutability clause enforced by the
ObservableList
returned byModel#updateFilteredAnswerableList
-
3.8. Proposed Features
3.8.1. Star Answerable Command
Overview of feature
User will be able to star an Answerable
during the test, which marks the Answerable
to remind them to revisit it after the test. The Answerable
will have an additional boolean
field star
.
The code flow follows the sequence diagram in section 3.2.3, but without the call to Answerable#isCorrect
.
The LogicManager
then calls execute(String)
in the reference frame "edit question as starred". This will involve the EditCommand
which is typically used in the configuration mode. It is now being called internally inside the quiz mode to update the Answerable
as starred.
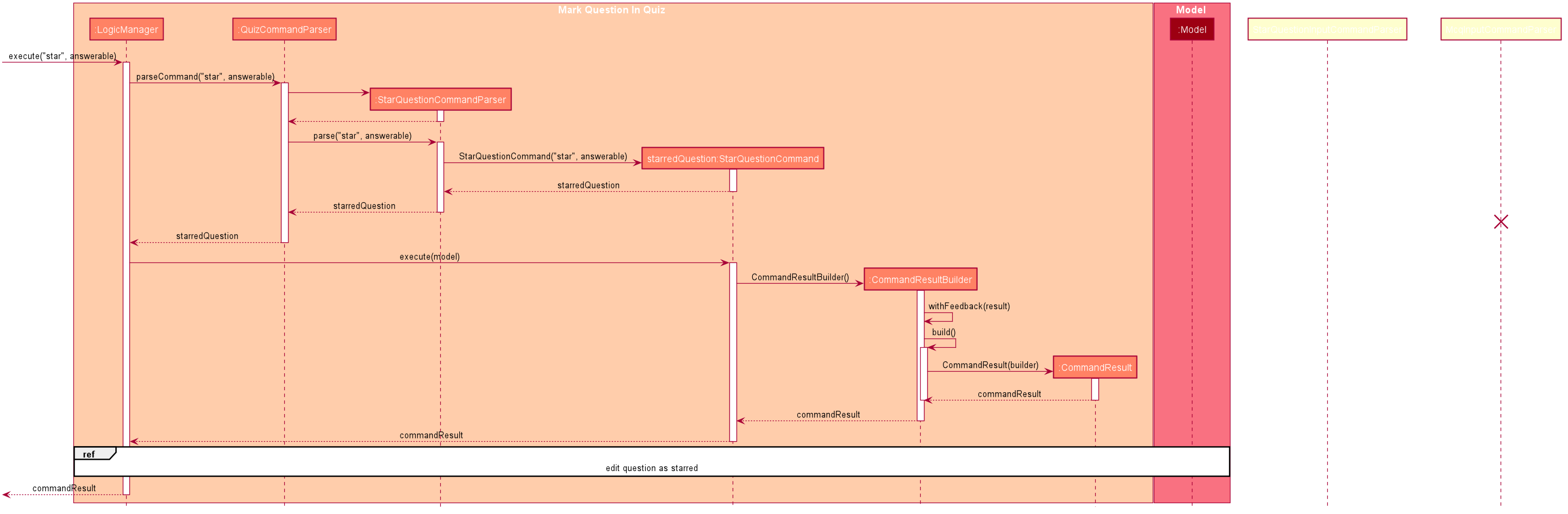
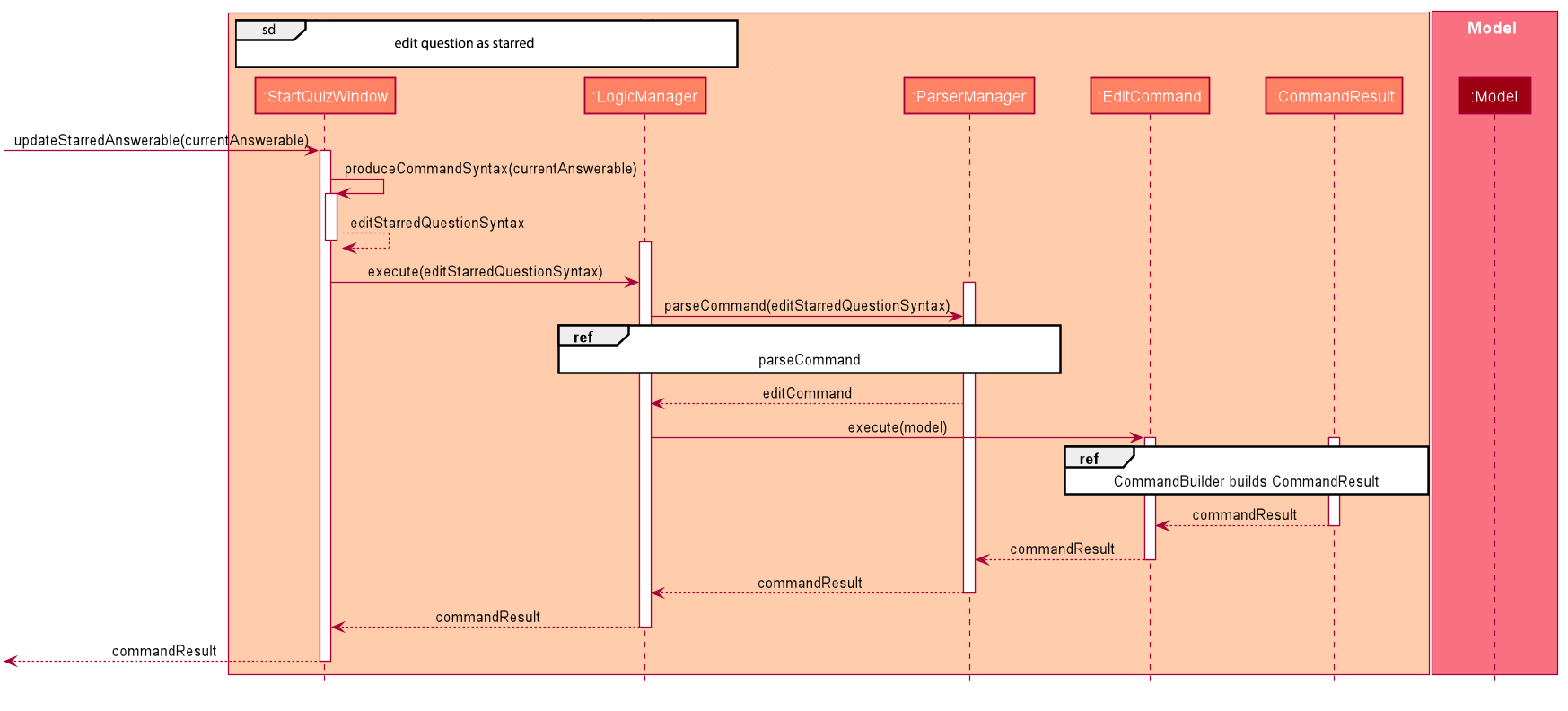
Answerable
as starred.Aspect: Updating the Answerable
to be marked as starred while inside quiz mode.
-
Alternative 1 (current choice): Update the
Answerable
through a call toLogicManager#execute
. This is primarily used during the configuration mode and not the quiz mode.-
Pro: Uses existing commands to implement a new feature for the user, appropriate code reuse
-
Con: No clear separation of logic as quiz mode should not know about configuration mode commands.
-
-
Alternative 2: Directly edit the
Answerable
as it is accessible in theexecute(String, Answerable)
for quiz mode commands.-
Pro: Less code needed.
-
Con: It breaks the implicit immutability of the
Answerable
, which should only be edited through theEditCommand
(which creates a newAnswerable
)
-
3.8.2. Input short code for quiz questions
Overview of feature
User will be able to input code, during the quiz session, and the RevisionTool
will check the syntax as the user types in the code.
The activity diagram is outlined below.
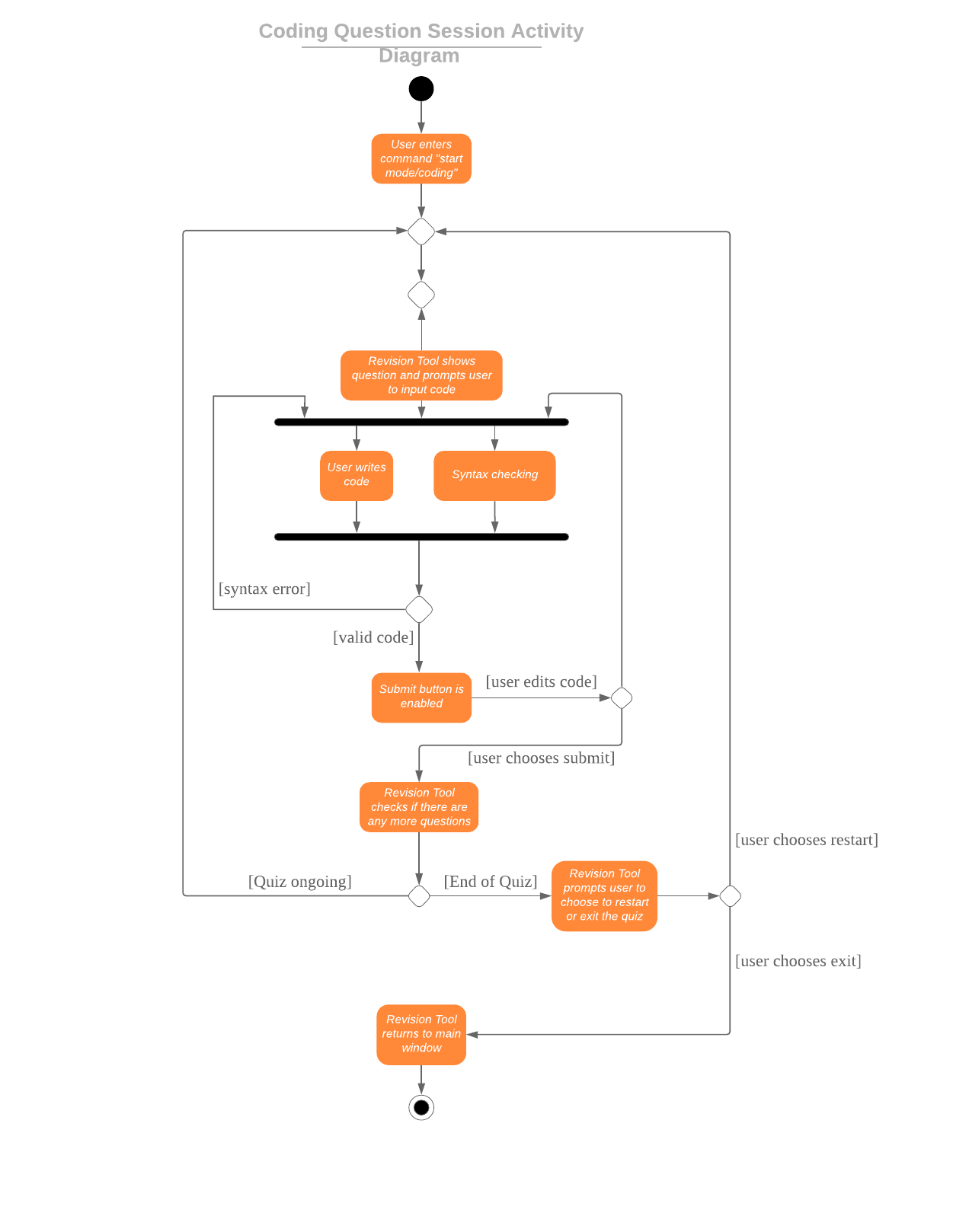
3.9. Logging
We are using java.util.logging
package for logging. The LogsCenter
class is used to manage the logging levels and logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file (See Section 3.10, “Configuration”) -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application -
WARNING
: Can continue, but with caution -
INFO
: Information showing the noteworthy actions by the App -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size
3.10. Configuration
Certain properties of the application can be controlled (e.g user prefs file location, logging level) through the configuration file (default: config.json
).
4. Documentation
Refer to the guide here.
5. Testing
Refer to the guide here.
6. Dev Ops
Refer to the guide here.
Appendix A: Product Scope
Target user profile:
-
is a CS2103/T student
-
prefer to use an app to help them to revise
-
can type fast
-
prefers typing over mouse input
-
is reasonably comfortable using CLI apps
Value proposition: helps student to ace CS2103/T
Appendix B: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
|
lazy CS2103 student |
refer to the revision tool solely for my consolidated module revision |
do not have to refer to Luminus |
|
CS2103 student |
have a personalised application to store all my questions and answers in one place |
refer to it conveniently for revision. |
|
CS2103 student |
have a revision tool to test my coding skills and concepts through writing short codes |
test myself on coding proficiency too. |
|
CS2103 student |
keep track and see how much progress I have made in completing the questions |
gauge my level of progress in completing the syllabus. |
|
vim-using CS2103/T student |
use the keyboard-based commands |
further increase my efficiency |
|
busy CS2103/T |
use quick revision tools |
learn using spaced-retrieval |
|
busy CS2103 student |
mark certain concepts as easy |
will not have to spend as much time studying the easy concepts. |
|
CS2103 student with a lot of things on my mind |
mark certain questions that I am unsure of |
refer back to the question when I am free. |
|
CS2103 student |
import questions from my peers |
study on my own. |
|
conscientious CS2103 student |
export the questions I am unsure of |
raise them up during tutorials. |
|
indecisive student |
be recommended questions instead of me having to plan my own study plan |
go directly to studying |
|
competitive CS2103 student |
at least know where I stand among my cohort |
look at who is the next person I can beat. |
|
gamer CS2103/T student |
accomplish tasks that give me a sense of achievement, preferably through in application rewards |
I feel good. |
|
A+ CS2103 student |
review and give suggestions to improve the application |
benefit more CS2103 students. |
|
CS2103 student |
port this application over to my other modules |
revise for my other modules using this application as well. |
|
unorganized CS2103 student |
get reminders about my quiz deadlines |
complete my quizzes on time |
|
organized CS2103 student |
schedule reminders to remind me when I should use the application to do revision |
will not forget to do revision. |
|
user of the application |
get an estimate of my final grade for CS2103 |
know what to expect on result release day. |
|
CS2103 peer tutor |
use this as a form of teaching tool |
teach better |
|
CAP 5.0 CS2103 student |
show off my IQ by perfecting my test scores |
motivate other students. |
|
CS2103 student |
view the questions/topics that most students answered wrongly |
revise for those topics. |
|
visual oriented student |
the app to have different colours as compared to the regular black and white |
learn better |
|
non-motivated CS2103 student |
use the application to remind me to study |
I will study |
|
student that wants shortcuts |
type a partial command and have it be auto-completed |
I can save time. |
|
CS2103 student new to Git |
have a help function which lists all the commonly used Git commands |
become more proficient with Git. |
|
master software engineer taking CS2103 |
be able to access the source code |
to make the application better and customise it for myself. |
|
CS2103 student |
get recommended a list of questions that I frequently get wrong |
learn from my mistakes |
|
lonely CS2103 student |
have someone to talk to, even if it’s a computer |
I won’t feel lonely |
|
CS2103 student who keeps having stomach ache |
the application to tell me where the nearest toilet is |
go and shit |
Appendix C: Use Cases
(For all use cases below, the System is the RevisionTool
and the Actor is the user
, unless specified otherwise)
Use case (UC01): Add answerable
MSS
-
User requests to add an answerable
-
RevisionTool adds the answerable
Use case ends.
Extensions
-
2a. The answerable already exists in the list.
-
2a1. RevisionTool shows an error message.
Use case ends.
-
-
2a. Any of the parameters provided are invalid.
-
2a1. RevisionTool shows an error message.
Use case ends.
-
Use case (UC02): Delete answerable
MSS
-
User requests to list answerables
-
RevisionTool shows a list of answerables
-
User requests to delete a specific answerable in the list
-
RevisionTool deletes the answerable
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. RevisionTool shows an error message.
Use case resumes at step 2.
-
Use case (UC03): Start Quiz in Normal Mode
MSS
-
User requests to start quiz in Normal Mode
-
RevisionTool shows the Quiz Mode window with the first question displayed.
-
User enters his input into the command box.
-
RevisionTool checks the input and records the result of the answer (i.e. whether correct or not).
-
RevisionTool displays the next question. Use case resumes at step 3.
Use case ends.
Extensions
-
1a. The list of questions for the quiz is empty.
Use case ends.
-
3a. The given input is invalid.
-
3a1. RevisionTool shows an error message.
Use case resumes at step 3.
-
-
3c. User inputs an exit command.
Use case ends.
-
5a. The current level of the quiz has ended.
-
5a1. RevisionTool prompts the user to continue or end the quiz.
-
5a2. User chooses to proceed to the next level.
Use case resumes at step 5.
-
-
5b. The current level of the quiz has ended.
-
5b1. RevisionTool prompts the user to continue or end the quiz.
-
5b2. User chooses to end the quiz.
Use case ends.
-
-
5c. The entire quiz has ended.
-
5c1. RevisionTool prompts the user to restart or end the quiz.
-
5c2. User chooses to restart the quiz.
Use case ends resumes at step 2.
-
-
5d. The entire quiz has ended.
-
5d1. RevisionTool prompts the user to restart or end the quiz.
-
5d2. User chooses to end the quiz.
Use case ends.
-
-
*a. Time available to answer each question of the quiz runs out (30 seconds for Normal Mode).
-
*a1. RevisionTool marks the question as wrong.
Use case resumes at step 5.
-
For Custom Mode, the use case is the same except timer can be customised. For Arcade Mode, an extension 4a will be added. If the user gets the question wrong, the quiz will end and the User will be prompted to restart or end the quiz. |
Appendix D: Non Functional Requirements
-
RevisionTool should work on any mainstream OS as long as it has Java
11
or above installed. -
RevisionTool be able to hold up to 1000 questions without any significant reduction in performance for typical usage.
-
A user with above slow typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
-
RevisionTool should be able to run without any internet connectivity.
-
RevisionTool does not require any further installation upon downloading the jar file.
Appendix F: Instructions for Manual Testing
Given below are instructions to test the app manually.
These instructions only provide a starting point for testers to work on; testers are expected to do more exploratory testing. |
F.1. Launch and Shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file
Expected: Shows the GUI with a set of sample answerables. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window by using the
x
button at the top right hand corner or by using the commandexit
. -
Re-launch the app by double clicking the jar file.
Expected: The most recent window size and location is retained.
-
F.2. Adding an answerable
-
Adding an MCQ to the current list.
-
Test case:
add type/mcq q/What is 1 + 1 y/2 x/1 x/3 x/4 cat/easy diff/1
Expected: new MCQ answerable will be created and appended at the bottom of the list. Details of the the added answerable will be shown at the bottom of the list, and the correct answer will be highlighted in green.
-
-
Adding a True False to the current list
-
Test case:
add type/tf q/What is 1 + 1 = 2 y/true cat/easy diff/1
Expected: new True False answerable will be created and appended at the bottom of the list. Details of the added answerable will be shown at the bottom of the list, and only the correct answer will be shown and highlighted in green.
-
-
Adding a Short Answer Question (SAQ) to the current list
-
Test case:
add type/saq q/What is smaller than 10 but bigger than 7? y/8 y/9 cat/easy diff/1
Expected: new SAQ answerable will be created and appended at the bottom of the list. Details of the added answerable will be shown at the bottom of the list and all the correct answers state will be highlighted in green.
-
-
Adding an Answerable that already exist in the Revision Tool
-
Test case:
add type/mcq q/What is 1 + 1 y/2 x/1 x/3 x/4 cat/easy diff/1
Expected: No new answerable will be added as the question already exist in the Revision Tool. An error message will be thrown, informing users that the answerable already exist in the Revision Tool.
-
F.3. Deleting an answerable
-
Deleting an answerable while all answerables are listed.
-
Prerequisites: List all answerables using the
list
command. Multiple answerables in the list. -
Test case:
delete 1
Expected: First answerable is deleted from the list. Details of the deleted answerable shown in the status message. Timestamp in the status bar is updated. -
Test case:
delete 0
Expected: No answerable is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
delete
,delete x
(where x is larger than the list size) {give more}
Expected: Similar to previous.
-
F.4. Starting quiz
-
Starting a quiz in various modes.
-
Test case:
start mode/normal
Expected: Start quiz window will pop up with a question showing under the command box and the answers in the result box further below. Answer the questions using the CLI accordingly to see the progress bar move till quiz completion. Users will be prompted if he wishes to proceed to level 2. -
Test case:
start mode/arcade
Expected: Start quiz window will pop up similar to previous test case. The only difference would be that once the quiz proceeds and an incorrect answer is input, the quiz ends and the score will be shown. This is the "hard mode" of our quiz mode. -
Test case
start mode/custom timer/3
Expected: Start quiz window will pop up similar to previous test case. The difference here will be the timer. Instead of the 30 seconds timer per question, the new timer (seen at the bottom right beside the status progress bar) will be at 3 seconds as set by the user.
-
F.5. Answering questions during Normal Mode
-
Answering an MCQ.
-
Test case:
a
Expected: Revision Tool will progress to the next question if there are still questions available.
-
-
Answering a True & False Question.
-
Test case:
t
Expected: Revision Tool will progress to the next question if there are still questions available.
-
-
Answering an SAQ.
-
Test case:
Short answer
Expected: Revision Tool will progress to the next question if there are still questions available.
-
-
Ending the quiz.
-
Test case:
exit
Expected: Revision Tool will end the quiz and go back to configuration mode.
-
For the scenarios 1-3. If the level has ended, the user will be prompted to continue to the next level or end the quiz. If the entire quiz has ended, the user will be prompted to restart or end the quiz. |
Testing for Custom Mode is similar to Normal Mode except that the category, difficulty and timer are customisable. For Arcade Mode, whenever the user answers a questions wrongly, the quiz will end and the user will be prompted to restart or end the quiz.
F.6. Saving data
-
Dealing with missing/corrupted data files
-
If there is a missing answerables data file, the RevisionTool will automatically create a default data file with all the default answerables inside.
-
If there is a corrupted answerable data file, the RevisionTool will automatically start with a list of empty file. Users will then be able to use the
#restoreCommand
here to get a list of default answerables or alternatively, create a new set of answerables manually.
-
-
To identify missing/corrupted data files:
-
.\data\revisiontool.json
-
.\data\history.json
-